The design of the hexapod looks like this. Each “limb” comprises of a shoulder, elbow and a foot. The shoulder moves in a horizontal plane, left and right. The elbow and feet move in vertical plans, up and down.
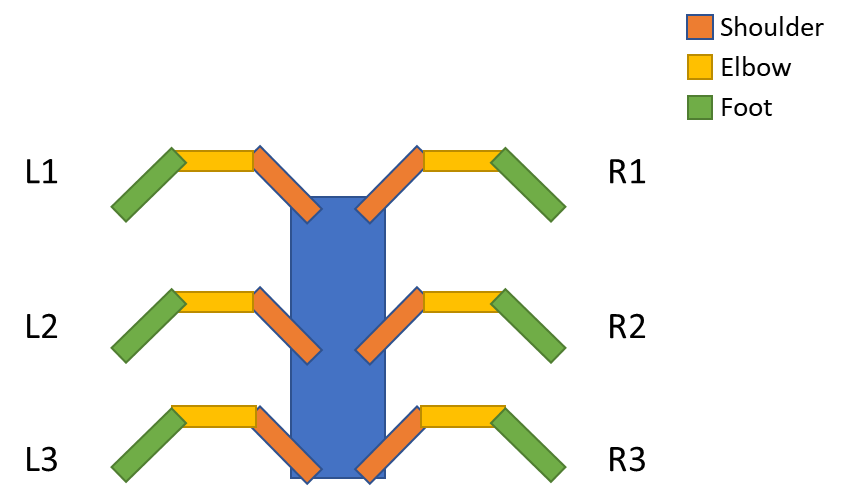
To control the hexapod, I need to consider stability. My current plan is to move three legs at a time. Something like this
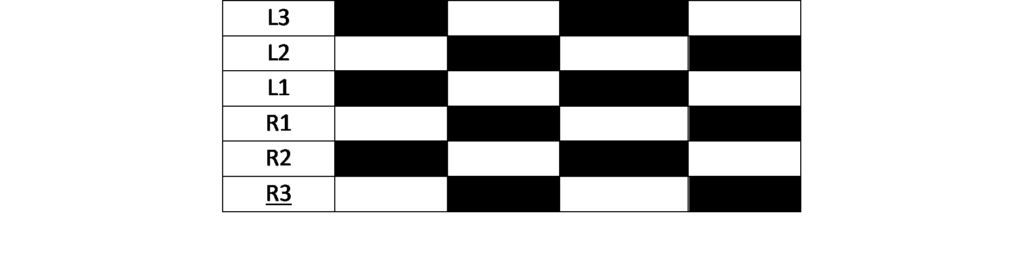
This is an example gait I’ve found online. It resembles how an ant walks. So either side always has at least one foot on the ground and the robot should be balanced. And this should be quicker (and easier) than moving each foot at a time.
#Problem – how do I make walking asynchronous? If I have a single function to move a limb, how do I move two limbs at once?
This is an example gait I’ve found online. It resembles how an ant walks. So either side always has at least one foot on the ground and the robot should be balanced. And this should be quicker (and easier) than moving each foot at a time.
#Problem – how do I make walking asynchronous? If I have a single function to move a limb, how do I move two limbs at once?
#Answer
/* Sweep
by BARRAGAN <http://barraganstudio.com>
This example code is in the public domain.
modified 8 Nov 2013
by Scott Fitzgerald
http://www.arduino.cc/en/Tutorial/Sweep
*/
#include <Servo.h>
Servo servo1; // create servo object to control a servo
Servo servo2; // create servo object to control a servo
// twelve servo objects can be created on most boards
int pos = 90; // variable to store the servo position
void setup()
{
servo1.attach(8); // attaches the servo on pin 9 to the servo object
servo2.attach(7); // attaches the servo on pin 9 to the servo object
}
void loop()
{
for(pos = 90; pos >= 19; pos --){
servo1.write(pos);
servo2.write(180-pos);
}
//delay(5000);
for(pos = 19; pos <= 90; pos ++) // goes from 19 degrees to 90 degrees
{
servo1.write(pos); // tell servo to go to position in variable 'pos'
servo2.write(180-pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15ms for the servo to reach the position
}
}
Note that this is my design process as I try and plan how I’m going to make this work, and part of that is trying to understand what’s possible. So this isn’t my code, but an example of how to control the servos I found here .